Use the test runner you like best!
The only thing your test runner needs to do is to produce TAP output on console.log or process.stdout. Here's an example project using mocha.
You can also use tape to output TAP output in browsers and in node. Here is what a simple tape test looks like:
var test = require('tape'); var myCode = require('../my_code.js'); test('make sure my code works', function (t) { t.plan(2); t.equal(myCode.beep(5), 555); myCode.boop(333, function (n) { t.equal(n, 3); }); });
This code is run through browserify so you can run commonjs-style tests written for node and even published to npm.
Just make sure all the files your test will need are checked into git so that browserify can find them. All the dependencies specified in your `package.json` will be installed with npm so your tests can depend on npm modules in addition to files you've placed directly in your git repo.
If you use tape, make sure to add it to the `devDependencies` field of your `package.json` like so:
{ "name": "my-code", "version": "0.1.4", "devDependencies": { "tape": "~2.3.0" }, "testling": { "files": "test/*.js", "browsers": [ "ie8", "ie9", "ff/13", "chrome/20" ] } }
You'll need to add a "testling" field to a `package.json` file if your project doesn't already have one. Testling-ci looks for 4 keys in the "testling" field: "files", "scripts", "browsers", and "harness". Here is an example `package.json` that uses "files":
{ "name": "pretty-great-module", "version": "0.0.0", "devDependencies": { "tape": "~2.3.0" }, "testling": { "files": "test/*.js", "browsers": { "ie": [ 8, 9 ], "firefox": [ 13 ], "chrome": [ 20 ], "safari": [ 5.1 ], "opera": [ 12 ] } } }
The "files" field is an array or string of file globs which determine the commonjs-style test files to be run. Each file in "files" gets browserified so you can `require()` libraries with a node-style module system.
Each file in the "files" field should output its results as TAP output with `console.log()`.
Here's an example "files" array of globs:
"files": [ "test/*.js", "blarg/**/*.js", "beep/boop.js" ]
If you have more tranditional, non-commonjs-style javascript, use the "scripts" field instead of "files". Each file in "scripts" has the same globbing behavior as "files", but each file is merely placed into a <script> tag on the page instead of being compiled with browserify.
Here's an example of the "scripts" field:
"scripts": [ "scripts/**/*.js", "tests/*.js", "vendor/test/robot_test.js" ]
Each file in the "scripts" field should output its results as TAP output with `console.log()`.
The "browsers" field is an object mapping browser names to arrays of browser versions. Here is an example:
"browsers": { "ie": [ 8, 9 ], "ff": [ 12, 13 ] "chrome": [ 20 ], "safari: [ 5.1 ] }
The "browsers" field may also be an array of strings using an optional "/" to separate browser names from versions. Here is an example of a "browser" field as an array:
"browsers": ["ie8","ie9","ff/12","chrome/20"]
For some test harnesses you can have a "harness" field. The "harness" field sets up test runners that are otherwise tricky to get running with TAP output. Here are the currently supported harnesses:
- "mocha-bdd" - mocha with --ui bdd
- "mocha-tdd" - mocha with --ui tdd
- "mocha-qunit" - mocha with --ui qunit
- "mocha-exports" - mocha with --ui exports
- "mocha" - alias for "mocha-bdd"
If you specify dependencies in the "dependencies" or "devDependencies" flags, they will be installed with npm and each test file will be bundled with browserify before being executed in the chosen browsers.
To configure your github open source repository to use testling-ci, visit:
https://github.com/USER/PROJECT/settings/hooks
then under the "WebHook URLs" add
as a URL. The next time you `git push` new commits, your tests will be run in the latest commit!
If you would like to use testling-ci with your private github repos or with repos not even hosted on github, please contact us to enroll in our TESTLING-CI PROFESH EDITION beta program.
You can let others know which browsers your library works with by displaying a badge on the project readme with the current state of your browser tests and a link to the test status page.
In your `readme.markdown` file, put:
[](https://ci.testling.com/USER/PROJECT)
Your readme should now contain an image like this:

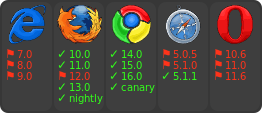